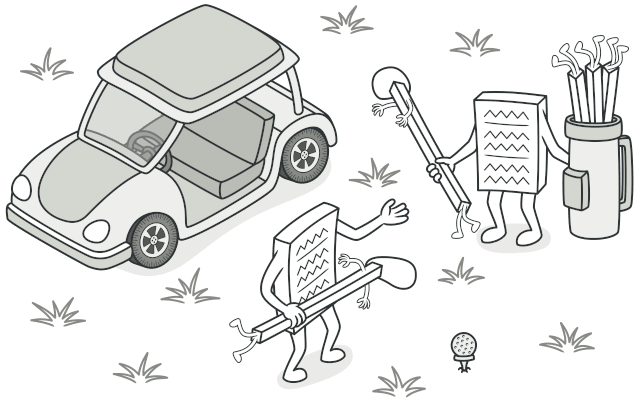
Strategy Pattern là gì
Strategy Pattern là một mẫu thiết kế hành vi (behavioral design pattern) trong lập trình hướng đối tượng. Mẫu thiết kế này cho phép bạn định nghĩa một tập hợp các thuật toán, đóng gói từng thuật toán vào các đối tượng riêng biệt và làm cho chúng có thể thay thế cho nhau.
Cài đặt Strategy Pattern
Các thành phần tham gia Strategy Pattern:
- Strategy : định nghĩa các hành vi có thể có của một Strategy.
- ConcreteStrategy : cài đặt các hành vi cụ thể của Strategy.
- Context : chứa một tham chiếu đến đối tượng Strategy và nhận các yêu cầu từ Client, các yêu cầu này sau đó được ủy quyền cho Strategy thực hiện.
// Strategy Interface
interface SortingStrategy {
void sort(int[] arr);
}
// Concrete Strategies
class BubbleSort implements SortingStrategy {
public void sort(int[] arr) {
// Sắp xếp mảng bằng thuật toán Bubble Sort
// ...
}
}
class QuickSort implements SortingStrategy {
public void sort(int[] arr) {
// Sắp xếp mảng bằng thuật toán Quick Sort
// ...
}
}
// Context
class Sorter {
private SortingStrategy sortingStrategy;
public Sorter(SortingStrategy sortingStrategy) {
this.sortingStrategy = sortingStrategy;
}
public void setSortingStrategy(SortingStrategy sortingStrategy) {
this.sortingStrategy = sortingStrategy;
}
public void sortArray(int[] arr) {
sortingStrategy.sort(arr);
}
}
// Sử dụng
public class Main {
public static void main(String[] args) {
int[] array = {5, 2, 9, 1, 5, 6};
Sorter sorter = new Sorter(new BubbleSort());
sorter.sortArray(array); // Sắp xếp mảng bằng Bubble Sort
sorter.setSortingStrategy(new QuickSort());
sorter.sortArray(array); // Sắp xếp mảng bằng Quick Sort
}
}
Tham khảo: