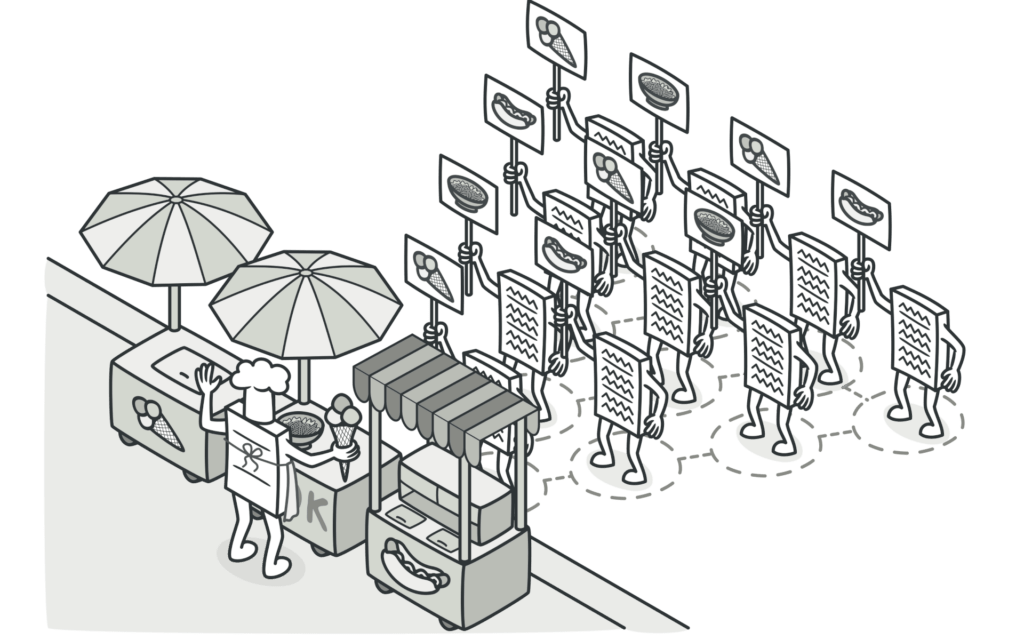
Visitor Pattern là gì
Visitor Pattern là một trong những Pattern thuộc nhóm hành vi (Behavior Pattern).
Cho phép bạn thêm các phương thức hoặc hành vi mới cho các lớp đối tượng mà không cần thay đổi cấu trúc của chúng.
Cài đặt Visitor Pattern
Cơ bản, Visitor Pattern bao gồm hai phần chính: các lớp đối tượng (object structure) và lớp visitor.
- Các lớp đối tượng định nghĩa cấu trúc của các đối tượng và thường có một phương thức chấp nhận các visitor.
- Lớp visitor chứa các phương thức để thăm các đối tượng trong cấu trúc. Mỗi phương thức của lớp visitor tương ứng với một loại đối tượng cụ thể trong cấu trúc và thực hiện các hành động cụ thể trên đối tượng đó.
interface Shape {
void accept(ShapeVisitor visitor);
}
// Đối tượng Circle
class Circle implements Shape {
private int radius;
public Circle(int radius) {
this.radius = radius;
}
public int getRadius() {
return radius;
}
@Override
public void accept(ShapeVisitor visitor) {
visitor.visit(this);
}
}
// Đối tượng Rectangle
class Rectangle implements Shape {
private int width;
private int height;
public Rectangle(int width, int height) {
this.width = width;
this.height = height;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
@Override
public void accept(ShapeVisitor visitor) {
visitor.visit(this);
}
}
// Visitor Interface
interface ShapeVisitor {
void visit(Circle circle);
void visit(Rectangle rectangle);
}
// Lớp visitor cụ thể thực hiện các hành động trên các đối tượng
class AreaCalculator implements ShapeVisitor {
private double totalArea;
public double getTotalArea() {
return totalArea;
}
@Override
public void visit(Circle circle) {
// Tính diện tích hình tròn
totalArea += Math.PI * circle.getRadius() * circle.getRadius();
}
@Override
public void visit(Rectangle rectangle) {
// Tính diện tích hình chữ nhật
totalArea += rectangle.getWidth() * rectangle.getHeight();
}
}
// Ví dụ sử dụng
public class Main {
public static void main(String[] args) {
Shape[] shapes = { new Circle(5), new Rectangle(3, 4), new Circle(2) };
AreaCalculator areaCalculator = new AreaCalculator();
for (Shape shape : shapes) {
shape.accept(areaCalculator);
}
System.out.println("Tổng diện tích của các hình: " + areaCalculator.getTotalArea());
}
}
Link tham khảo: