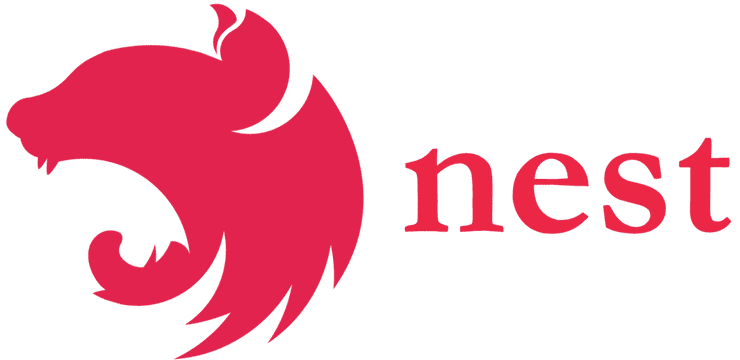
Mở Đầu Giới Thiệu
NestJS là một framework Node.js mạnh mẽ, được xây dựng dựa trên TypeScript và lấy cảm hứng từ các khái niệm của Angular. NestJS giúp các nhà phát triển tạo ra các ứng dụng server-side có cấu trúc rõ ràng, dễ bảo trì và mở rộng. Với khả năng hỗ trợ tuyệt vời cho TypeScript, NestJS mang lại trải nghiệm lập trình hiện đại, mạnh mẽ và hiệu quả. Trong bài viết này, chúng ta sẽ khám phá cách khởi tạo một project bằng Nest CLI và xây dựng một REST API cơ bản với NestJS.
Khởi Tạo Project Bằng Nest CLI
1. Cài Đặt Nest CLI
Trước tiên, bạn cần cài đặt Nest CLI, công cụ giúp bạn khởi tạo và quản lý các project NestJS một cách dễ dàng.
$ npm install -g @nestjs/cli
2. Tạo Project Mới
Sử dụng lệnh nest new
để tạo một project NestJS mới:
$ nest new my-nest-project
Bạn sẽ được yêu cầu chọn công cụ quản lý package (npm hoặc yarn). Sau khi chọn và hoàn thành quá trình cài đặt, di chuyển vào thư mục project:
$ cd my-nest-project
Viết REST API Bằng NestJS
1. Tạo Module và Controller
NestJS sử dụng các module để tổ chức mã nguồn. Mỗi module chứa các thành phần như controller, service, và các thành phần khác liên quan.
- Tạo một module và controller cho “articles”:
bash $ nest generate module articles $ nest generate controller articles $ nest generate service articles
2. Định Nghĩa Model
Sử dụng TypeScript để định nghĩa một model cho Article. Tạo một file article.model.ts
trong thư mục src/articles
và thêm mã sau:
export class Article {
id: number;
title: string;
body: string;
}
3. Viết Service
Service chịu trách nhiệm xử lý logic nghiệp vụ. Mở file src/articles/articles.service.ts
và thêm mã sau:
import { Injectable } from '@nestjs/common';
import { Article } from './article.model';
@Injectable()
export class ArticlesService {
private articles: Article[] = [];
private idCounter = 1;
findAll(): Article[] {
return this.articles;
}
findOne(id: number): Article {
return this.articles.find(article => article.id === id);
}
create(article: Article): Article {
article.id = this.idCounter++;
this.articles.push(article);
return article;
}
update(id: number, articleData: Partial<Article>): Article {
const article = this.findOne(id);
if (!article) return null;
Object.assign(article, articleData);
return article;
}
delete(id: number): void {
this.articles = this.articles.filter(article => article.id !== id);
}
}
4. Viết Controller
Controller định nghĩa các tuyến đường và xử lý các yêu cầu HTTP. Mở file src/articles/articles.controller.ts
và thêm mã sau:
import { Controller, Get, Post, Body, Param, Put, Delete } from '@nestjs/common';
import { ArticlesService } from './articles.service';
import { Article } from './article.model';
@Controller('articles')
export class ArticlesController {
constructor(private readonly articlesService: ArticlesService) {}
@Get()
findAll(): Article[] {
return this.articlesService.findAll();
}
@Get(':id')
findOne(@Param('id') id: number): Article {
return this.articlesService.findOne(id);
}
@Post()
create(@Body() article: Article): Article {
return this.articlesService.create(article);
}
@Put(':id')
update(@Param('id') id: number, @Body() articleData: Partial<Article>): Article {
return this.articlesService.update(id, articleData);
}
@Delete(':id')
delete(@Param('id') id: number): void {
this.articlesService.delete(id);
}
}
5. Đăng Ký Module
Đảm bảo rằng ArticlesModule
được đăng ký trong AppModule
. Mở file src/app.module.ts
và thêm ArticlesModule
:
import { Module } from '@nestjs/common';
import { ArticlesModule } from './articles/articles.module';
@Module({
imports: [ArticlesModule],
})
export class AppModule {}
Kết Luận
NestJS cung cấp một framework mạnh mẽ và linh hoạt cho việc phát triển backend với Node.js. Bằng cách sử dụng Nest CLI và các công cụ tích hợp, bạn có thể nhanh chóng khởi tạo và xây dựng các ứng dụng REST API một cách dễ dàng và hiệu quả. Hy vọng rằng qua bài viết này, bạn đã có cái nhìn tổng quan về cách sử dụng NestJS để xây dựng backend. Hãy bắt tay vào thực hiện và khám phá thêm nhiều tính năng tuyệt vời mà NestJS mang lại!