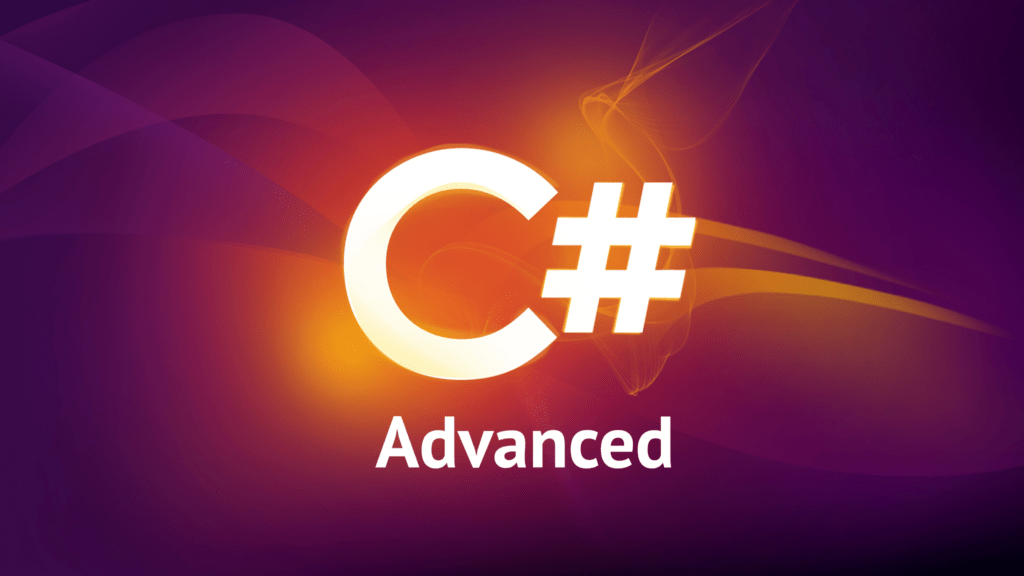
- C# – Anonymous Types
- C# – Dynamic Types
- C# – Ternary Operator ?:
- C# Generics & Generic Constraints
- C# – Delegates
- C# – Func Delegate
- C# – Action Delegate
- C# – Anonymous Method
- C# – Events
- C# – Extension Method
- C# – HttpClient
C# – Action Delegate
Action là một loại delegate được xác định trong System namespace. Action type delegate giống như Func delegate ngoại trừ việc Action delegate không trả về giá trị. Nói cách khác, bạn có thể sử dụng Action delegate với một phương thức có loại trả về void.
Ví dụ: delegate sau in ra một giá trị int.
// Example: C# Delegate public delegate void Print(int val); static void ConsolePrint(int i) { Console.WriteLine(i); } static void Main(string[] args) { Print prnt = ConsolePrint; prnt(10); } // OUTPUT: 10
Bạn có thể sử dụng Action delegate thay vì xác định Print delegate ở trên, ví dụ:
// Example: Action delegate static void ConsolePrint(int i) { Console.WriteLine(i); } static void Main(string[] args) { Action<int> printActionDel = ConsolePrint; printActionDel(10); } // OUTPUT: 10
Bạn có thể khởi tạo một Action delegate bằng từ khóa new hoặc bằng cách gán trực tiếp một phương thức:
Action<int> printActionDel = ConsolePrint; //Or Action<int> printActionDel = new Action<int>(ConsolePrint);
An Action delegate can take up to 16 input parameters of different types.
An Anonymous method can also be assigned to an Action delegate, for example:
// Example: Anonymous method with Action delegate static void Main(string[] args) { Action<int> printActionDel = delegate(int i) { Console.WriteLine(i); }; printActionDel(10); } // OUTPUT: 10
Một biểu thức Lambda cũng có thể được sử dụng với Action delegate:
// Example: Lambda expression with Action delegate static void Main(string[] args) { Action<int> printActionDel = delegate(int i) { Console.WriteLine(i); }; printActionDel(10); } // OUTPUT: 10
Do đó, bạn có thể sử dụng bất kỳ phương thức nào không trả về giá trị với các loại Action delegate.
Ưu điểm của Action and Func Delegates
- Dễ dàng và nhanh chóng để xác định các delegates.
- Làm cho mã ngắn.
- Khả năng tương thích type trong toàn bộ ứng dụng
Những điểm cần nhớ:
- Action delegate giống như func delegate ngoại trừ việc nó không trả về bất cứ thứ gì. Kiểu trả về phải trống.
- Action delegate có thể có 0 đến 16 tham số đầu vào
- Bạn có thể sử dụng Action delegate với anonymous methods hoặc lambda expressions.