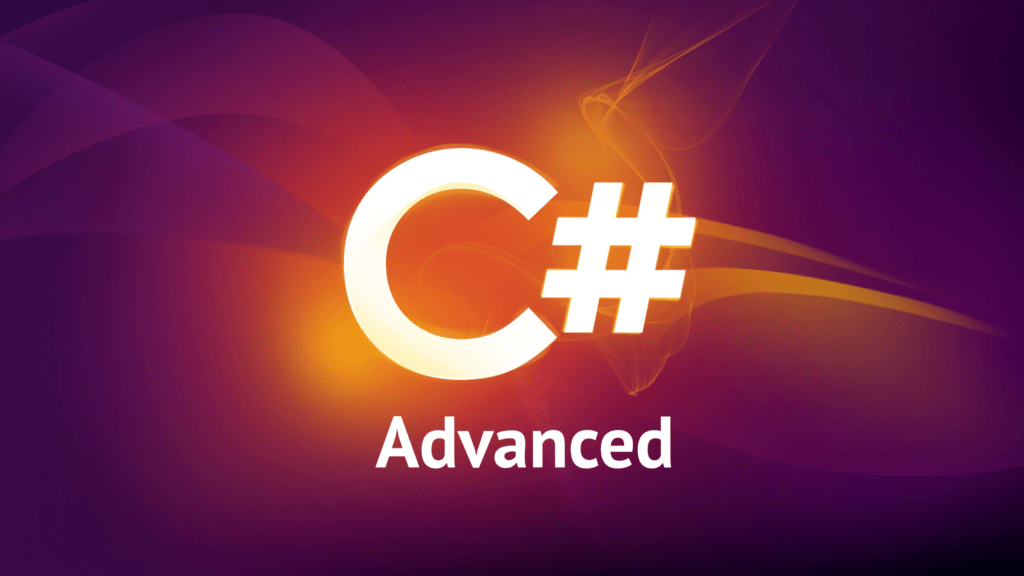
- C# – Anonymous Types
- C# – Dynamic Types
- C# – Ternary Operator ?:
- C# Generics & Generic Constraints
- C# – Delegates
- C# – Func Delegate
- C# – Action Delegate
- C# – Anonymous Method
- C# – Events
- C# – Extension Method
- C# – HttpClient
C# – Consume Web API in .NET using HttpClient
.NET 2.0 bao gồm class WebClient để giao tiếp với máy chủ web bằng HTTP protocol. Tuy nhiên, class WebClient có một số hạn chế. .NET 4.5 bao gồm class HttpClient để khắc phục hạn chế của WebClient.
Ở đây, chúng ta sẽ sử dụng class HttpClient trong console application để gửi và nhận dữ liệu từ Web API được lưu trữ trên máy chủ web IIS cục bộ.
Bạn cũng có thể sử dụng HttpClient trong các ứng dụng .NET khác như MVC web application, windows form application, windows service application, …
Hãy xem cách sử dụng Web API bằng HttpClient trong console application.
Chúng ta sẽ consume Web API sau được tạo trong phần trước..
// Example: Web API Controller using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Http; using System.Web.Http; namespace MyWebAPI.Controller { public class StudentController : ApiController { public IHttpActionResult GetAllStudents(bool includeAddress = false) { IList<StudentViewModel> students = null; using (var ctx = new SchoolDBEntities()) { students = ctx.Students.Include("StudentAddress").Select(s => new StudentViewModel() { Id = s.StudentID, FirstName = s.FirstName, LastName = s.LastName, Address = s.StudentAddress == null || includeAddress == false ? null : new AddressViewModel() { StudentId = s.StudentAddress.StudentID, Address1 = s.StudentAddress.Address1, Address2 = s.StudentAddress.Address2, City = s.StudentAddress.City, State = s.StudentAddress.State } }).ToList<StudentViewModel>(); } if (students.Count == 0) { return NotFound(); } return Ok(students); } public IHttpActionResult PostNewStudent(StudentViewModel student) { if (!ModelState.IsValid) return BadRequest("Not a valid data"); using (var ctx = new SchoolDBEntities()) { ctx.Students.Add(new Student() { StudentID = student.Id, FirstName = student.FirstName, LastName = student.LastName }); ctx.SaveChanges(); } return Ok(); } public IHttpActionResult Put(StudentViewModel student) { if (!ModelState.IsValid) return BadRequest("Not a valid data"); using (var ctx = new SchoolDBEntities()) { var existingStudent = ctx.Students.Where(s => s.StudentID == student.Id).FirstOrDefault<Student>(); if (existingStudent != null) { existingStudent.FirstName = student.FirstName; existingStudent.LastName = student.LastName; ctx.SaveChanges(); } else { return NotFound(); } } return Ok(); } public IHttpActionResult Delete(int id) { if (id <= 0) return BadRequest("Not a valid studet id"); using (var ctx = new SchoolDBEntities()) { var student = ctx.Students .Where(s => s.StudentID == id) .FirstOrDefault(); ctx.Entry(student).State = System.Data.Entity.EntityState.Deleted; ctx.SaveChanges(); } return Ok(); } } }
Step 1:
Đầu tiên, Tạo a console application trong Visual Studio 2013 cho Desktop.
Step 2:
Mở NuGet Package Manager console từ TOOLS -> NuGet Package Manager -> Package Manager.
Làm theo câu lệnh dưới đây:
Install-Package Microsoft.AspNet.WebApi.Client
Step 3:
Bây giờ, Tạo một Student model class bời vì chúng ta sẽ gửi và nhận Student object đến Web API của chúng ta.
// Example: Model Class public class Student { public int Id { get; set; } public string Name { get; set; } }
Send GET Request
Làm theo ví dụ gửi HTTP GET request đến Student Web API và hiển thị kết quả trên console.
// Example: Send HTTP GET Request using HttpClient using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Net.Http; using System.Net.Http.Headers; namespace HttpClientDemo { class Program { static void Main(string[] args) { using (var client = new HttpClient()) { client.BaseAddress = new Uri("http://localhost:60464/api/"); //HTTP GET var responseTask = client.GetAsync("student"); responseTask.Wait(); var result = responseTask.Result; if (result.IsSuccessStatusCode) { var readTask = result.Content.ReadAsAsync<Student[]>(); readTask.Wait(); var students = readTask.Result; foreach (var student in students) { Console.WriteLine(student.Name); } } } Console.ReadLine(); } } }
Hãy hiểu ví dụ trên từng bước một.
Đầu tiên, chúng tôi đã tạo một object của HttpClient và đã chỉ định địa chỉ Web API. Phương thức GetAsync() gửi một http GET request tới url được chỉ định.
Phương thức GetAsync() là một async method và trả về một Task. Task.wait() tạm dừng việc thực hiện cho đến khi GetAsync() phương thức hoàn thành thực thi và trả về kết quả.
Khi mà việc thực thi hoàn thành, Chúng ta lấy kết quả từ Task sử dụng Task.result đó là kiểu HttpResponseMessage.
Bây giờ, bạn can kiểm tra trạng thái http response sử dụng IsSuccessStatusCode. Đọc nội dung của kết quả sử dụng ReadAsAsync() method.
Vì vậy, bạn có thể gửi http GET request sử dụng HttpClient object và xử lý kết quả.
Send POST Request
Tương tự, bạn có thể gửi yêu cầu HTTP POST bằng phương thức PostAsAsync() của HttpClient và xử lý kết quả theo cách tương tự như yêu cầu GET.
Ví dụ sau gửi yêu cầu http POST tới Web API của chúng tôi. Nó post đối tượng Sinh viên dưới dạng json và nhận được phản hồi.
// Example: Send HTTP POST Request using HttpClient using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Net.Http; using System.Net.Http.Headers; namespace HttpClientDemo { class Program { static void Main(string[] args) { var student = new Student() { Name = "Steve" }; var postTask = client.PostAsJsonAsync<Student>("student", student); postTask.Wait(); var result = postTask.Result; if (result.IsSuccessStatusCode) { var readTask = result.Content.ReadAsAsync<Student>(); readTask.Wait(); var insertedStudent = readTask.Result; Console.WriteLine("Student {0} inserted with id: {1}", insertedStudent.Name, insertedStudent.Id); } else { Console.WriteLine(result.StatusCode); } } } }
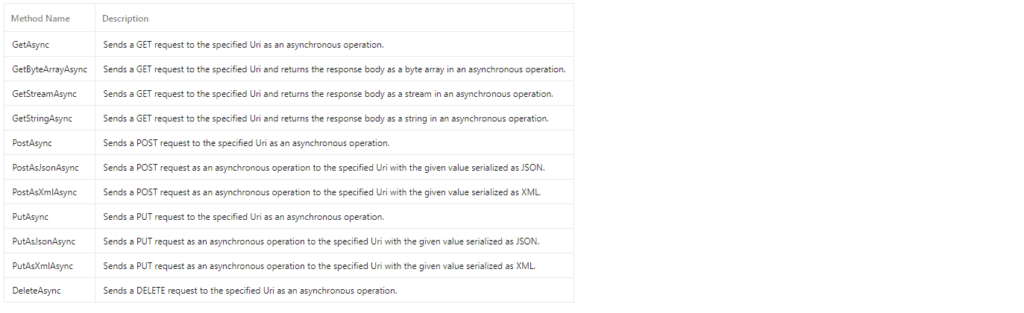
Visit MSDN to know all the members of HttpClient[https://msdn.microsoft.com/en-us/library/system.net.http.httpclient(v=vs.118).aspx ] and HttpClientExtension[https://msdn.microsoft.com/en-us/library/system.net.http.httpclientextensions(v=vs.118).aspx ].